This comprehensive guide aims to walk you through the process of building an iOS app with React Native. We will cover everything from setting up your development environment, creating your first project, to deploying your app on the Apple App Store.
![]() |
Image by StockSnap from Pixabay |
Understanding React Native
React Native is an open-source JavaScript framework developed by Facebook. It allows developers to build mobile applications using JavaScript and React, a popular JavaScript library for building user interfaces. Unlike other JavaScript frameworks that render via WebView, React Native communicates directly with the platform, which means your React Native app will render using real mobile UI components.
Why Choose React Native for Your iOS App Development?
React Native is a fantastic choice for developing iOS apps for several reasons:
- Write Once, Use Everywhere: React Native enables you to write code once and deploy it on both iOS and Android platforms. This can save a significant amount of time and resources compared to developing separate applications for each platform.
- Familiar Syntax: If you're already accustomed to React and JavaScript, you'll feel right at home with React Native. Even if you're new to React, it's relatively easy to grasp, and there are numerous resources available to help you get started.
- Live Reloading: React Native allows you to see the effect of changes in your code in real-time, which facilitates rapid development and debugging.
- Community Support: React Native has a robust community around it, with a plethora of libraries and tools available to help you develop your application.
Setting Up Your React Native Environment for iOS
Before you can start building your React Native iOS app, there are a few tools and software that you'll need to get set up:
- Xcode: Xcode is Apple's IDE (Integrated Development Environment) for both Mac and iOS apps. It's where you'll build and run your React Native iOS application. Xcode is free and available for download in the Mac App Store.
- Node.js and npm: Node.js is a JavaScript runtime that lets you run JavaScript on your server or your machine, while npm (Node Package Manager) is a package manager for Node.js. React Native uses Node.js to build your JavaScript code, and npm to manage React Native dependencies.
- React Native CLI: The React Native CLI (Command Line Interface) is a tool that lets you create new React Native apps. You can install it globally on your machine using npm.
- Watchman: Watchman is a tool by Facebook for watching changes in the filesystem. It's highly recommended for better performance.
- CocoaPods: CocoaPods is a dependency manager for Swift and Objective-C Cocoa projects. It has over 74 thousand libraries and is used in over 3 million apps.
To install these tools, you can use the following commands:
# Install Node.js and npm
brew install node
# Install Watchman
brew install watchman
# Install React Native CLI
npm install -g react-native-cli
# Install CocoaPods
sudo gem install cocoapods
Once these tools are installed, you're ready to start creating your React Native project.
![]() |
Image by fsweb_de from Pixabay |
Creating Your First React Native Project
To create a new React Native project, you can use the react-native init
command followed by the name of your project:
react-native init MyFirstApp
This command creates a new directory with the given name (MyFirstApp in this case), with all the setup needed for a basic React Native application.
Developing Your React Native iOS App
Now that you have a new React Native project set up, you can start developing your iOS app. Open the App.js
file in your project's root directory, and you can start writing your React Native code.
React Native components are very similar to React components. All React APIs are also available in React Native, so if you're familiar with React, you'll have no trouble getting started with React Native.
Here's a simple example of a React Native component:
import React, { useState } from 'react';
import { Button, SafeAreaView, StatusBar, View, Text, StyleSheet } from 'react-native';
const App = () => {
const [count,setCount] = useState(0);
return (
<SafeAreaView>
<StatusBar/>
<View style={styles.view}>
<Button onPress={()=>setCount(count+1)} title={`Count is ${count}`} />
</View>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
view: {
marginTop: "100%",
},
});
export default App;
This simple app displays a button with a count that increments each time the button is pressed.
Testing Your React Native iOS App
Once you've written your app, you can run it using the react-native run-ios
command from your project directory. This will start a local server for your app and open it in an iOS simulator.
react-native run-ios
You should now see your app running in the iOS simulator. Try clicking the button to increment the count.
Making Network Calls
One of the most common tasks in mobile apps is to communicate with external services. React Native provides a uniform abstraction over platform APIs for making network calls. Here's an example of making a network request to a random dog image API and displaying the resulting image:
import React, { useEffect, useState } from "react";
import { ActivityIndicator, SafeAreaView, Text, View, Image } from "react-native";
function App() {
const [isLoading, setLoading] = useState(true);
const [data, setData] = useState(null);
useEffect(() => {
fetch("https://random.dog/woof.json")
.then((response) => response.json())
.then((json) => setData(json.url))
.catch((error) => console.error(error))
.finally(() => setLoading(false));
}, []);
return (
<View style={{ flex: 1, padding: 24 }}>
<SafeAreaView />
{isLoading ? <ActivityIndicator /> : <Image source={{uri: data}} style={{width: 200, height: 200}}/>}
</View>
);
}
export default App;
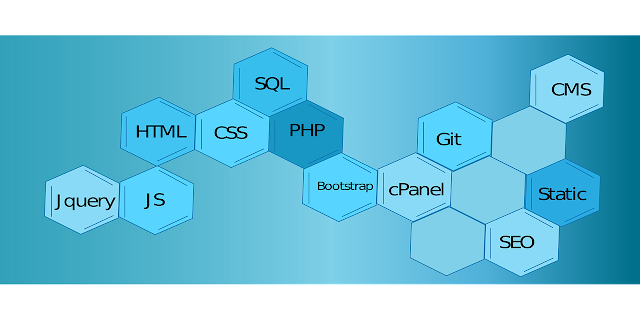
Image by airraya from Pixabay
Building Your React Native iOS App for Production
Before you can distribute your React Native app, you'll need to build it for production. This process involves setting up your project for distribution, configuring your app's settings, creating a distribution build, and testing it.
First, you'll need to configure your app for distribution. This involves setting the correct bundle identifier and version number, setting up code signing, and configuring other settings in Xcode.
Next, create a distribution build of your app. You can do this by selecting the "Archive" option from the "Product" menu in Xcode.
Finally, test your distribution build. You can do this by installing the build on a device or using TestFlight, Apple's testing service.
Deploying Your React Native iOS App to the App Store
Once your app is built and tested, you can submit it to the App Store. This involves uploading your app to App Store Connect, filling out the necessary metadata and screenshots, and submitting your app for review.
After your app is reviewed and approved by Apple, it will be available for download on the App Store.
Conclusion
React Native is a powerful tool for building cross-platform mobile applications. With it, you can build an iOS app using JavaScript, leveraging the power and flexibility of the React library. This guide has shown you how to set up your development environment, create a new project, write your React Native code, and deploy your app to the App Store.
Building an iOS app with React Native may seem complex at first, but once you understand the basics, you'll find that it's a highly efficient and effective way to build mobile apps. Happy coding!